What is a ReplicaSet
ReplicaSet is a Kubernetes object which ensures that a specified number of pods are running at any given time. The limitation with Pods is that they are short lived and cannot automatically reschedule itself if the Pod or the node hosting the pod fails. As a result, users will no longer be able to access the application. To prevent users from losing access to our application we would like to have more than one instance of pod running at the same time. That way even if one of the pods fail, we still have our application running on the other one.
The ReplicaSet helps us to run multiple instances of a single pod in the Kubernetes cluster thus providing High Availability. This does not mean that ReplicaSet cannot be used if you wish to run a single instance of the pod. Even if you have a single pod, ReplicaSet can help by automatically creating a new pod when the existing one fails thus ensuring that the specified number of pods are running at all time.
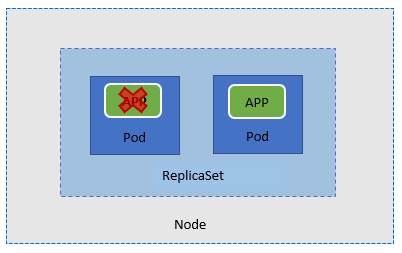
Why to use ReplicaSet
- Load Balancing – As ReplicaSet ensures that the specified number of pods are running, traffic can be equally sent across the pods.
- Scaling – With ReplicaSet we can quickly scale the application by adding or removing pod.
- High Availability – The ReplicaSet helps us to run multiple instances of a single pod in the Kubernetes cluster thus providing High Availability.
Configuring a ReplicaSet
Just like any other resource, the ReplicaSet manifest file consist of four main sections as below.
apiVersion:
kind:
metadata:
[ . . . ]
spec:
replicas:
selector:
[ . . . ]
template:
[ . . . ]
- apiVersion: Defines the Kubernetes API that supports ReplicaSet.
- kind: Specifies the Kubernetes object we wish to create.
- metadata: Contains information about the ReplicaSet object.
- We can specify name and labels associated with the ReplicaSet.
- spec: There are three sections under spec namely replicas, selector and template.
- replicas: Specifies the number of replicas of the pod
- selector: The selector is used to identify which pod the ReplicaSet is responsible for.
- template: Defines the pod template that the ReplicaSet uses when creating pods and adding them to meet the required number of replicas.
Let create a manifest file for the ReplicaSet.
apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: frontend
labels:
app: webserver
tier: frontend
spec:
replicas: 3
selector:
matchLabels:
tier: frontend
template:
metadata:
labels:
tier: frontend
spec:
containers:
- name: webserver
image: nginx
When the above manifest file is applied to the Kubernetes cluster it will
- Create a ReplicaSet with the name
frontend
as specified undermetadata
. - Create a pod with label “
tier: frontend
” as specified underspec.template.metadata.labels
- Three replicas of the pod as specified under
spec.replicas
- The selector will manage pods with labels “
tier: frontend
” as specified underspec.selector.matchLabels
Apply the manifest file to the Kubernetes cluster and verify the status of the ReplicaSet deployed.
# kubectl apply -f replicaSet.yml
replicaset.apps/frontend created
# kubectl get rs
NAME DESIRED CURRENT READY AGE
frontend 3 3 3 11s
- NAME: lists the names of the ReplicaSet.
- DESIRED: displays the desired number of replicas of the application. This is the desired state.
- CURRENT: displays how many replicas are currently running.
- READY: displays how many replicas of the application are available.
- AGE: displays how long the application has been running.
To get detailed information of the ReplicaSet
# kubectl describe rs/frontend
Name: frontend
Namespace: default
Selector: tier=frontend
Labels: app=webserver
tier=frontend
Annotations: <none>
Replicas: 3 current / 3 desired
Pods Status: 3 Running / 0 Waiting / 0 Succeeded / 0 Failed
Pod Template:
Labels: tier=frontend
Containers:
webserver:
Image: nginx
Port: <none>
Host Port: <none>
Environment: <none>
Mounts: <none>
Volumes: <none>
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal SuccessfulCreate 27s replicaset-controller Created pod: frontend-24mk5
Normal SuccessfulCreate 27s replicaset-controller Created pod: frontend-2bbq5
Normal SuccessfulCreate 27s replicaset-controller Created pod: frontend-rghpz
And lastly you can check for the Pods created by the ReplicaSet. You can verify that the ownerReferences of the Pod is set to ReplicaSet named frontend.
# kubectl get pods
NAME READY STATUS RESTARTS AGE
frontend-rghpz 1/1 Running 0 34s
frontend-2bbq5 1/1 Running 0 34s
frontend-24mk5 1/1 Running 0 34s
# kubectl get pods frontend-rghpz -o yaml
apiVersion: v1
kind: Pod
metadata:
creationTimestamp: "2023-01-05T20:34:23Z"
generateName: frontend-
labels:
tier: frontend
name: frontend-rghpz
namespace: default
ownerReferences:
- apiVersion: apps/v1
blockOwnerDeletion: true
controller: true
kind: ReplicaSet
name: frontend
uid: 19e75316-b90a-4c5e-91b1-cf1937c490ec
Scaling a ReplicaSet
A ReplicaSet can be scaled up or down by simply updating the value of replicas. To scale up, set the value of replicas to a higher number and apply the manifest file to the Kubernetes scale.
apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: frontend
labels:
app: webserver
tier: frontend
spec:
replicas: 5
selector:
matchLabels:
tier: frontend
template:
metadata:
labels:
tier: frontend
spec:
containers:
- name: webserver
image: nginx
# kubectl apply -f replicaSet.yml
replicaset.apps/frontend configured
# kubectl get rs
NAME DESIRED CURRENT READY AGE
frontend 5 5 5 9m41s
# kubectl get pods
NAME READY STATUS RESTARTS AGE
frontend-rghpz 1/1 Running 0 9m42s
frontend-24mk5 1/1 Running 0 9m42
frontend-p2gvh 1/1 Running 0 9m43s
frontend-n6cjd 1/1 Running 0 3s
frontend-dz4gt 1/1 Running 0 3s
Similarly to scale down the application set the value of replicas to a lower number. Scaling up or down can also be done via command line. For example, to scale down to two replicas run the below command.
# kubectl scale --replicas=2 rs/frontend
replicaset.apps/frontend scaled
# kubectl get rs
NAME DESIRED CURRENT READY AGE
frontend 2 2 2 14m
# kubectl get pods
NAME READY STATUS RESTARTS AGE
frontend-rghpz 1/1 Running 0 14m
frontend-24mk5 1/1 Running 0 14m
Alternatives to ReplicaSet
Deployment (recommended)
Deployment is an object which can own ReplicaSets and provides some more features over ReplicaSet. When you use Deployments, you don’t have to worry about managing the ReplicaSets that they create. Deployments own and manage their ReplicaSets. As such, it is recommended to use Deployments when you want to use ReplicaSets. In addition to that Deployment also provides features like rolling update in which Pods can be updated one by one ensuring zero downtime of the application. In addition, Deployment also support rollback, pause and resume of the upgrade.
DaemonSet
Use a DaemonSet instead of a ReplicaSet for Pods that provide a machine-level function, such as logging or collecting metrics. DaemonSet ensures that a replica of Pod is running on all the nodes of the cluster. In this case there is no need to specify the replica count. These pods are tied to the machine lifetime.
Job
Use a Job instead of a ReplicaSet for Pods that are expected to terminate on their own (that is, batch jobs).
Final Thoughts
In this post, we showed you how to use Kubernetes ReplicaSets to achieve High availability and automate scaling of application for better traffic handling. We also discussed other alternative resources – including Deployments, Job, and DaemonSets – and explained their use case.
More
Learn about Pods
Learn about Deployment
Pingback: Kubernetes: What is a Deployment? – Learn and Implement DevOps